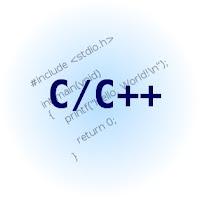
This blog post is continued from Apache log4cxx framework – Part One
This post comprises of a simple Configuration file and how it can be incorporated to a c++ code file for using the log4cxx framework.
Add the following simple xml Configuration file and name it as “MyLog4CxxConfig.xml”
<?xml version="1.0" encoding="UTF-8" ?>
<log4j:configuration xmlns:log4j="http://jakarta.apache.org/log4j/">
<appender name="ConsoleAppend" class="org.apache.log4j.ConsoleAppender">
<param name="Target" value="System.out"/>
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="%-5p %c{1} - %m%n"/>
</layout>
</appender>
<appender name="LogFileAppend" class="org.apache.log4j.FileAppender">
<param name="file" value="MyLogFile.log" />
<param name="append" value="true" />
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="%d %5p %c{1} - %m%n" />
</layout>
</appender>
<root>
<priority value="all" />
<appender-ref ref="ConsoleAppend"/>
</root>
<category name="TestFunction" >
<priority value ="info" />
<appender-ref ref="LogFileAppend" />
</category>
</log4j:configuration>
The above configuration is very simple one and it is supposed to output all the log messages to the console as the Log level is set to “all” and “info” level log messages will be appended to “MyLogFile.log”.
Now add “Test.cpp” to your project. This is the simple threaded example for testing log4cxx. Include the following header files ‘pthread.h’, ‘sched.h’, ‘log4cxxlogger.h‘, ‘log4cxxxmldomconfigurator.h’
using namespace log4cxx;
using namespace log4cxx::xml;
using namespace log4cxx::helpers;
LoggerPtr MyMainLogger(Logger::getLogger("TestFunction"));
void* TestFunction(void* arg){
LOG4CXX_INFO(MyMainLogger, "Test Function Started!");
int data = (int) arg;
char thread_data[10];
sprintf(thread_data, "thread - %d", data);
LOG4CXX_INFO(MyMainLogger, thread_data);
LOG4CXX_INFO(MyMainLogger, "Test Function Completed!");
}
int main(int argc, char** argv[])
{
DOMConfigurator::configure("MyLogConfig.xml");
int my_data, thread_num = 5;
pthread_t thread_id[thread_num];
for (int i = 0; i < thread_num; i++) {
my_data = i;
if (pthread_create(&(thread_id[i]), NULL, TestFunction, (void *) my_data) == 0) {
} else {
LOG4CXX_ERROR(MyMainLogger, "pthread create error.");
exit(1);
}
sched_yield();
}
for (int i = 0; i < thread_num; i++) {
pthread_join(thread_id[i], NULL);
}
return 0;
}
The above Test.cpp creates 5 posix threads which executes the TestFunction. When using this sample, please add PThreads Static Library from Netbeans Project properties or add the following line of code “-lpthread” to LDLIBSOPTIONS in the make debug file.
Very helpful. Thanks.