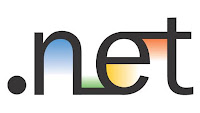
To convert an Image from one format to another, use the following Method.
Here, the known formats include, Bmp, Emf, Exif, Gif, Icon, Jpeg, Png, Tiff, Wmf etc.
First, include the following Namespaces;
using System.IO.Path;
using System.Drawing.Imaging;
using System.Drawing.Imaging;
Now assume you have Tiff image file in the following directory,
C:/Temp/myImage.tif
Assume you want to convert it to png and save it in the same directory, just use the below line of code in your project.
ConvertImageFormat(“C:/Temp/myImage.tif”, ImageFormat.Png)
The implementation of the above method is given below,
private bool ConvertImageFormat(String imagepath, ImageFormat format)
{
bool status = false;
string newfile;
+ GetFileNameWithoutExtension(imagepath) + “.”
+ format.ToString();
obj.Dispose();
status = true;
}
catch(SystemException) {
}
return status;
}
string newfile;
try {
Bitmap obj = new Bitmap(imagepath);
newfile = GetDirectoryName(imagepath) + “\”
+ GetFileNameWithoutExtension(imagepath) + “.”
+ format.ToString();
obj.Save(newfile, format);
obj.Dispose();
status = true;
}
catch(SystemException) {
}
return status;
}