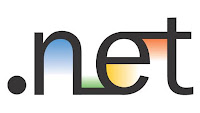
Assume you have a XML file with the following format.
<paramlist><param><key>1</key><value>100</value></param>
<param><key>2</key><value>150</value></param></paramlist>
Include the following Namespaces
<param><key>2</key><value>150</value></param></paramlist>
Include the following Namespaces
using System.Collections.Generic;
using System.Xml.Linq;
using System.Xml.Linq;
Assume your requirement is to read data from XML file and use the data in your project for further requirement. Include the below method in your code block to convert the XML Data to Dictionary.
GetDictionary(GetXmlDocument(Server.MapPath(“TempXml.xml”)))
Implementation of the above methods are given below,
private XDocument GetXmlDocument(string myPath) {
try {
XDocument xdocument = XDocument.Load(myPath);
return xdocument;
}
catch (Exception) {
throw new Exception(“XML file could not be loaded”);
}
}
}
}
public Dictionary GetDictionary(XDocument xdoc)
{
{
Dictionary dictionary = new Dictionary();
int count = xdoc.Elements().Descendants(“param”).Count();
int count = xdoc.Elements().Descendants(“param”).Count();
try{
for (int i = 0; i < count; i++)
{
Double key = Double.Parse(xdoc.Elements().Descendants(“key”).ElementAt(i).Value);
Double value =
{
Double key = Double.Parse(xdoc.Elements().Descendants(“key”).ElementAt(i).Value);
Double value =
Double.Parse(xdoc.Elements().Descendants(“value”).ElementAt(i).Value);
dictionary.Add(key, value);
}
dictionary.Add(key, value);
}
}
return dictionary;
}
}